How to Remove Duplicate characters from String in C#
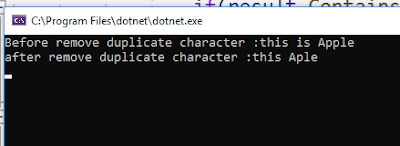
Hello All, In this Program, I will show you, how to make a program to remove duplicate characters from strings using c# using System; using System.Collections.Generic; namespace testconsole { class Program { static void Main(string[] args) { string Stringvalue = "this is Apple"; string result = ""; Console.WriteLine("Before remove duplicate character :{0} ", Stringvalue); foreach (char ch in Stringvalue) { if(result.Contains(ch.ToString())) { } else { result = result + ch; } } Console.WriteLine("after remove duplicate character :{0} ", result); Console.ReadKey(); } } }